Avatar
Setup
<script>
import { Avatar } from 'svelte-5-ui-lib';
</script>
Interactive Avatar Bilder
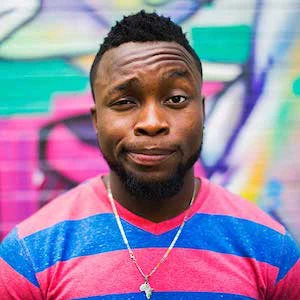
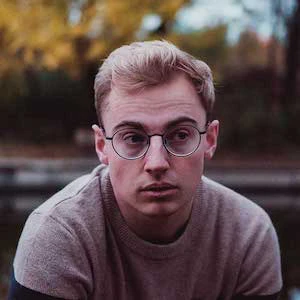
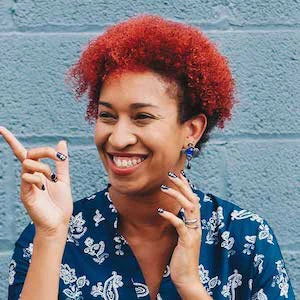
<Avatar />
Avatar text
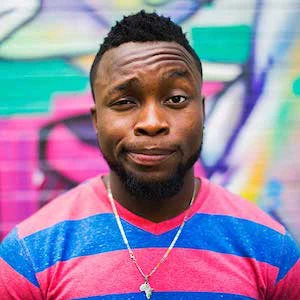
Jese Leos
Joined in August 2014
<script lang="ts">
import { Avatar } from 'svelte-5-ui-lib';
</script>
<div class="flex items-center space-x-4 rtl:space-x-reverse">
<Avatar src="/images/profile-picture-1.webp" alt="Profile picture 1" cornerStyle="rounded" />
<div class="space-y-1 font-medium dark:text-white">
<div>Jese Leos</div>
<div class="text-sm text-gray-500 dark:text-gray-400">Joined in August 2014</div>
</div>
</div>
Dot indicator
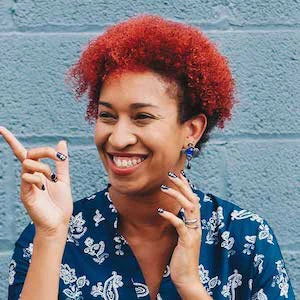
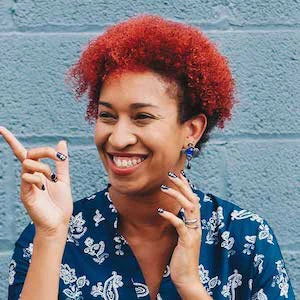
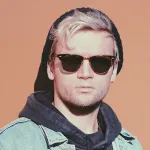
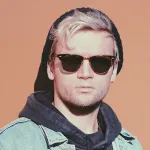
<script lang="ts">
import { Avatar } from 'svelte-5-ui-lib';
</script>
<div class="flex justify-center gap-4">
<Avatar src="/images/profile-picture-3.webp" alt="Profile picture 1" dot={{ color: 'red' }} />
<Avatar
src="/images/profile-picture-3.webp"
alt="Profile picture 2"
dot={{ placement: 'top-right', color: 'red' }}
cornerStyle="rounded"
/>
<Avatar
src="/images/profile-picture-5.webp"
alt="Profile picture 3"
dot={{ placement: 'bottom-right', color: 'green' }}
/>
<Avatar
src="/images/profile-picture-5.webp"
alt="Profile picture 4"
dot={{ placement: 'bottom-right' }}
cornerStyle="rounded"
/>
<Avatar dot={{}} />
</div>
Placeholder
<script lang="ts">
import { Avatar } from 'svelte-5-ui-lib';
</script>
<div class="flex justify-center gap-4">
<Avatar alt="placeholder" />
<Avatar cornerStyle="rounded" alt="rounded placeholder" />
<Avatar border alt="bordered placeholder" />
<Avatar cornerStyle="rounded" border alt="rounded bordered placeholder" />
</div>
Placeholder initial
JL
<script lang="ts">
import { Avatar } from 'svelte-5-ui-lib';
</script>
<div class="flex h-10 justify-center">
<Avatar alt="JL">JL</Avatar>
</div>
Stacked
<script lang="ts">
import { Avatar } from 'svelte-5-ui-lib';
</script>
<div class="flex flex-col justify-center gap-4 px-4">
<div class="mb-5 flex">
<Avatar src="/images/profile-picture-1.webp" stacked alt="Profile picture 1" />
<Avatar src="/images/profile-picture-2.webp" stacked alt="Profile picture 2" />
<Avatar src="/images/profile-picture-3.webp" stacked alt="Profile picture 3" />
<Avatar stacked />
</div>
<div class="flex">
<Avatar src="/images/profile-picture-1.webp" stacked alt="Profile picture 4" />
<Avatar src="/images/profile-picture-2.webp" stacked alt="Profile picture 5" />
<Avatar src="/images/profile-picture-3.webp" stacked alt="Profile picture 6" />
<Avatar
stacked
href="/"
class="bg-gray-700 text-sm text-white hover:bg-gray-600"
alt="Profile picture 7">+99</Avatar
>
</div>
</div>
User dropdown
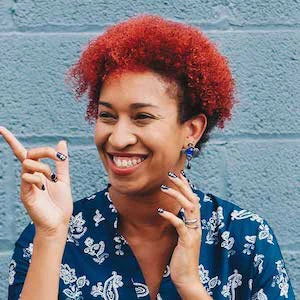
<script lang="ts">
import {
Avatar,
Dropdown,
DropdownHeader,
DropdownUl,
DropdownLi,
DropdownDivider,
uiHelpers
} from 'svelte-5-ui-lib';
let dropdown = uiHelpers();
let dropdownStatus = $state(false);
let closeDropdown = dropdown.close;
$effect(() => {
// this can be done adding nav.navStatus directly to DOM element
// without using effect
dropdownStatus = dropdown.isOpen;
});
</script>
<div class="flex h-64 items-start justify-center">
<Avatar
onclick={dropdown.toggle}
src="/images/profile-picture-3.webp"
alt="Profile picture"
class="cursor-pointer"
dot={{ color: 'green' }}
/>
<div class="relative">
<Dropdown {dropdownStatus} {closeDropdown} class="absolute top-[40px] -left-[100px]">
<DropdownHeader>
<div>Bonnie Green</div>
<div class="truncate font-medium">name@flowbite.com</div>
</DropdownHeader>
<DropdownUl>
<DropdownLi href="/">Dashboard</DropdownLi>
<DropdownLi href="/nav">Navbar</DropdownLi>
<DropdownLi href="/footer">Footer</DropdownLi>
<DropdownDivider />
<DropdownLi href="/dropdown">Dropdown</DropdownLi>
</DropdownUl>
</Dropdown>
</div>
</div>
Avatar with tooltip
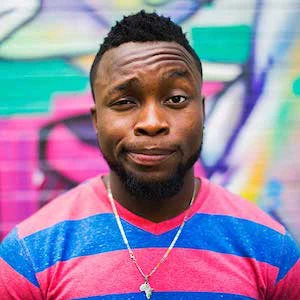
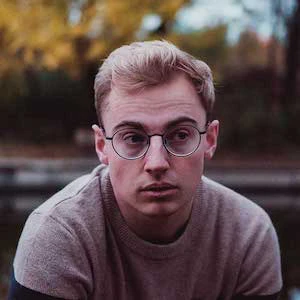
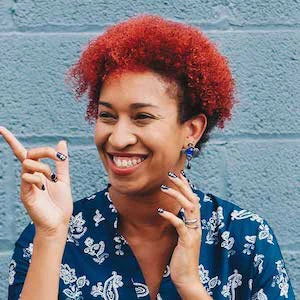
<script>
import { Avatar, Tooltip } from 'svelte-5-ui-lib';
</script>
<div class="flex h-32 items-center justify-center gap-8">
<Avatar id="jese" cornerStyle="rounded" alt="Jese Leos" src="/images/profile-picture-1.webp" />
<Tooltip triggeredBy="#jese">Jese Leos</Tooltip>
<Avatar
id="robert"
cornerStyle="rounded"
alt="Robert Gouth"
src="/images/profile-picture-2.webp"
/>
<Tooltip triggeredBy="#robert">Robert Gouth</Tooltip>
<Avatar
id="bonnie"
cornerStyle="rounded"
alt="Bonnie Green"
src="/images/profile-picture-3.webp"
/>
<Tooltip triggeredBy="#bonnie">Bonnie Green</Tooltip>
</div>